DevOps Consulting & Agile Training
Why DevOps & Agile
Why Choose SPR
Fostering a culture of continuous improvement is extremely important for long-term success in adopting DevOps.
“Part of that is creating an environment where individuals and teams can learn from each other and grow together. Organizational leaders must ensure that there are opportunities for this to happen and continue to support this type of collaboration.”
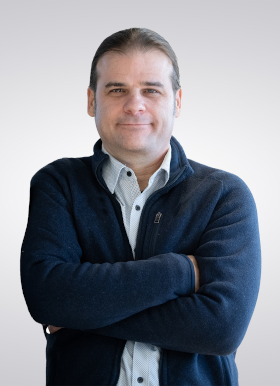
Modernizing a Consumer Behavior Data Co.
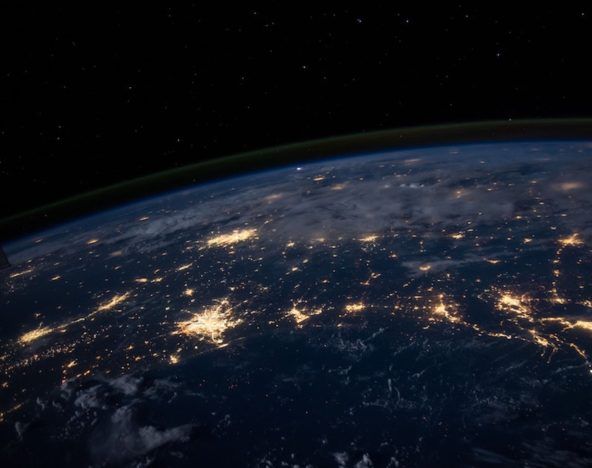
Modernizing a Consumer Behavior Data Co.
A leading global information and measurement company that studies consumer behavior recognized their development processes needed to be faster and more agile, in order to quickly create meaningful content and applications. They engaged SPR to assess whether their teams were ready to move from a more traditional development structure to the open culture of DevOps. We performed five assessments, creating roadmaps with detailed steps to create a more unified team and work structure. Several of the assessment findings focused on inefficiencies that could be remedied with modern tools and automation.
Featured Insights
Our thought leaders provide insight on industry news and trends in our Lumen magazine.