Enjoy the Vue: Rapid Prototyping to Create Proofs of Concept
When working in an agile environment, rapid prototyping can be a quick and effective way of creating proofs of concept and reusable components. This is particularly true when time is limited and you need near-immediate feedback from your stakeholders. In this article, we’ll look at specific features in Vue.js and Vue CLI 3.0 that help you easily go from ideas and concepts to code.
Vue.js
New to Vue? Here’s the breakdown: Vue.js is a Javascript UI/SPA framework and open-source project created by Evan You, a developer for Google. Vue.js was designed to be a lightweight, high-performance alternative to Angular.js. Released in early 2014, it quickly became popular with front-end developers. Since then it’s grown to be as popular and viable of a framework as React.js. As of the time of this writing, Vue.js has been favorited over React.js on Github by a margin of more than 4.5k stars. You can find more information about Vue along with installation instructions at https://vuejs.org.
The recent release of Vue CLI 3.0 gives us some exciting new features, as well as some already existing ones to help facilitate rapid prototyping. Some of those features are:
- Project management with Vue UI
- Hot module replacement
- Serving individual .vue components
- Mounting and Shallow Mounting components with Jest (For unit testing)
- Environmental Variables management
I recommend taking a moment to explore the Vue CLI tool so that you can see first hand everything that it offers.
Management
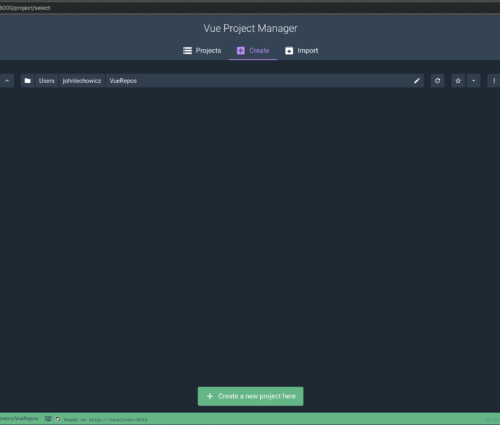
Vue UI (Beta) is one of the new features part of the new CLI 3.0 tool set. In lieu of using a command line, this gives the user a GUI for project management tasks such as:
- Project selection and creation
- Project tasks like serving and testing
- Node.js package management
As part of the Vue project creation process, you will be prompted to save your selected settings as a preset. That way, when creating a new project in the future, you can re-use the same project settings instead of manually configuring new projects each time.
To launch the Vue UI, simply open your terminal window and type:
> vue ui
After selecting a project you’ll see the following project management screen:
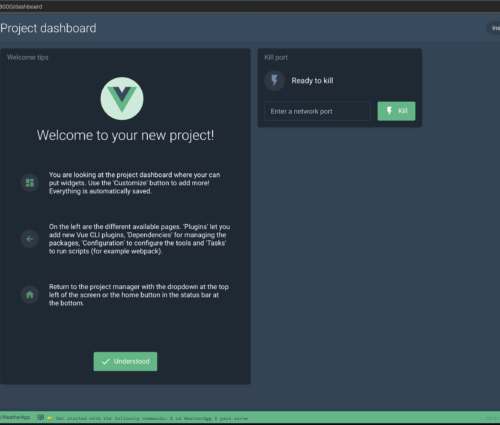
From here, let’s install dependencies via the UI.
First, click the “Dependencies” menu item. Then in the adjacent screen, enter the name of the node package you want to add. Click install. You also have the option of marking it as a dev dependency. That’s it!
Now, let’s look at the “Tasks” menu item. This is where we can serve the web app locally, build it, and run our unit tests.
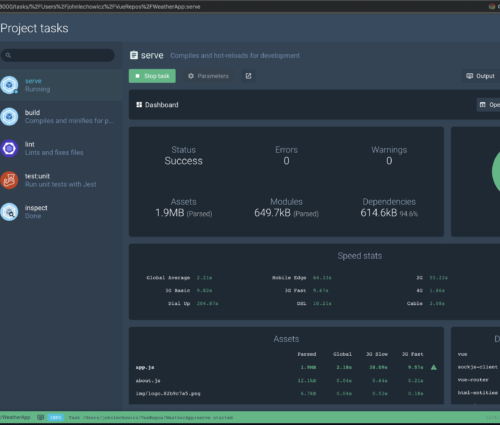
What’s great about the serve task is that it handles spinning up a dev web server for viewing the Vue app over localhost, which saves you time for working on your business logic. You can run and view the output of your unit tests here as well, proving it’s very handy to have the Vue UI running while working on your app.
Single Serving Components
The Vue CLI 3.0 tool makes it easy to mount and serve individual vue components without having to go through the trouble of creating an app to show the component. In your terminal, simply browse to the directory that has your component you want to mount, and type:
> vue serve my_component.vue
Where my_component.vue is the file name of the component you want to view.
The Vue CLI tool will then spin up a local server to display the component. This is fine if you simply want to get an idea of the component’s layout. There is one caveat, however. By default, Vue CLI will load only the component and nothing else, which includes CSS files and Javascript libraries. In order to be able to see and interact with your component prototype in a manner more consistent with it’s intent of use, an extra step is required here. My solution is to create a wrapper component for the prototype and import your CSS and JS files as necessary. Here is an example of my wrapper for a component I recently wrote:
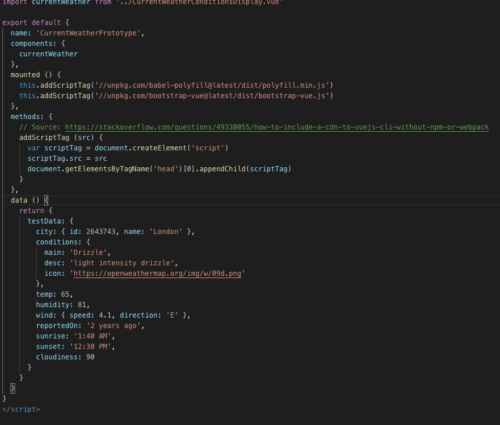
When I serve my wrapper component I get this:
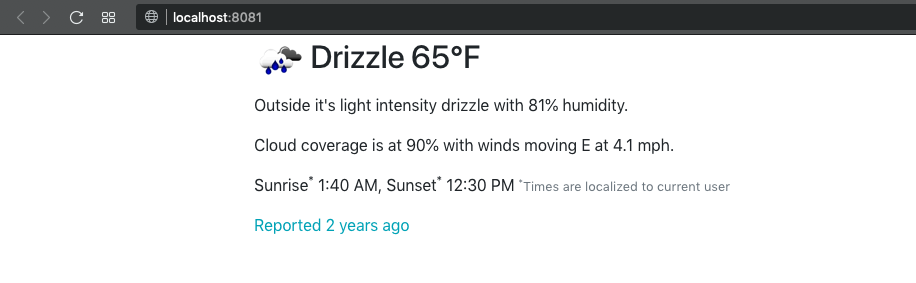
Having “Hot Module Reload” enabled will allow you to view your changes to either the wrapper or prototype component directly in the browser.
Mounting with Jest
If using Jest as your unit test framework, you can also write tests around individual components. The Vue.js test suite comes with two methods: “mount” and “shallowMount”. While both methods mount the given component, there is one small but significant difference:
- Using “mount” will cause the test framework to mount and render the given component and any child components defined within.
- Using “shallowMount” will render the given component ONLY. Any child components defined in your component will not be rendered.

While the test highlighted above is trivial, you can see the basic gist of it. You can write more in-depth tests using this method around your components output. Enabling “Watch files for changes…” will give you the added benefit of live testing whenever you update your components.
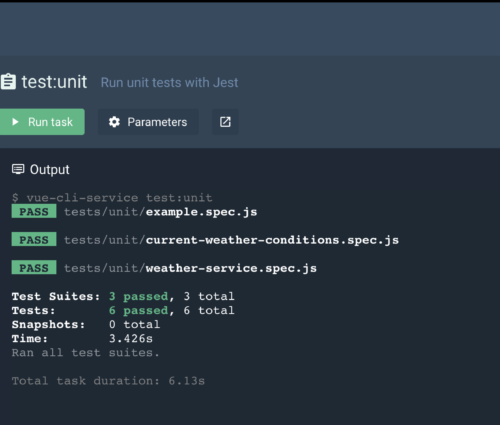
Environmental Variables
Working with environmental variables in Vue has been simplified with the CLI 3.0 release as well. All of your project’s environmental variables can be stored in a single “.env” file located in your project’s root. Name them with the prefix VUE_APP_
and the Vue cli serve and build commands will take care of initializing these values for you at runtime. More info on creating .env files for different environments (i.e production, staging, testing) can be found here: https://cli.vuejs.org/guide/mode-and-env.html#modes
Wrapping it up
Using rapid prototyping in your projects is something that definitely merits consideration. Note, some frameworks support the concept better than others. However, it may be just cause for you to reevaluate your current framework to see if it is meeting your project’s agile needs. In either case, it’s another strategy worth keeping in mind should you find yourself in a tough spot on any project.