Custom Software & Mobile App Development
Why Choose SPR's Custom Development Services
From modernizing old systems to building new applications from the ground up, our team is well-versed in providing custom software solutions that set your business up for success now and into the future. Our core expertise is B2B custom development for businesses of all sizes, including small, medium/large, and enterprise. We use cutting-edge technologies and platforms that cater to your unique needs, skill sets, and goals. And, our work spans many industries, including:
- Development for Insurance
- Development for Financial Services
- Development for Professional Services
- Development for Logistics Companies
- Development for Software Companies (SaaS)
- Development for B2B Companies
- Development for Manufacturing Companies
No matter the stakes, we build clean, well-tested code that uses the latest generation of browsers and platforms, and we ensure our solutions are secure, scalable, and maintainable to meet demands for volume and maximum processing, whether on premises or in the cloud. From languages and technology platforms, frameworks to protocols and best practices, such as Agile, DevOps, test-driven development, or continuous delivery, we develop custom software to meet your exact needs.
Our Core Services
Why Modernize Your Software
The biggest advantages to working with SPR: We’re unbiased when it comes to tools, platforms, and technologies. We do it all.
"Our buyers and clients are diverse, and our skillset complements that. Rather than choose the one tool we know the best and stretch its boundaries, we choose the best tool for the job—that versatility is a big win. Similarly, just as we can build something for you, we’re also open to building something with you and then mentoring your team on it. Our partners love that."
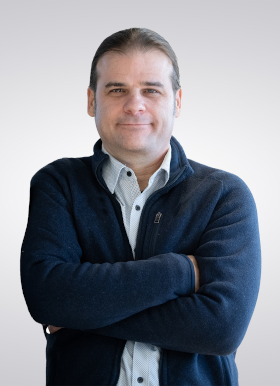
Custom Development Story: Streamlining Trade Compliance
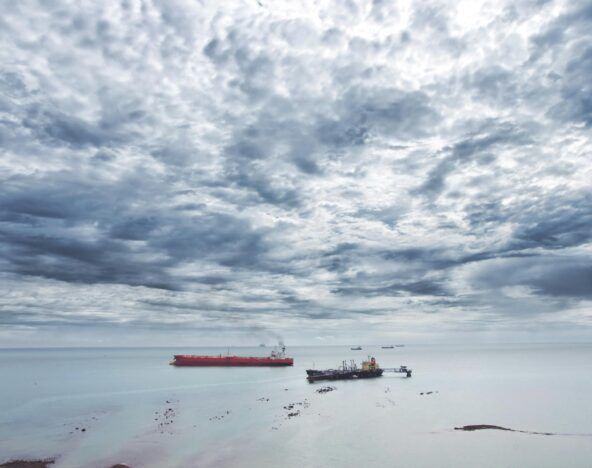
Custom Development Story: Streamlining Trade Compliance
Facing fragmented workflows and inconsistent reporting due to disparate systems, a leading customs brokerage and compliance company needed a unified platform to streamline its operations. SPR stepped in to develop a cloud-native application that integrated and automated critical processes such as product classification, trade agreement qualification, and post-entry audits. The result is a powerful, secure platform that simplifies trade compliance management and supports business growth.
Featured Insights
Our thought leaders provide insight on industry news and trends in our Lumen magazine.